C language is not domain specific. It is used to develop application software of different domains. This is the power as well as drawback of C language. It depends on the skills of programmers to implement programming constructs of C language.
Till now C language has been used to develop software like operating systems, database, compilers etc., Microsoft Windows, Linux, Mac, iOS, Android, Oracle, MySQL, 3D Movies, Embedded systems etc. Each of these software applications use and manipulate Data.
Data is information about an entity. Data is defined by similar or different fields. If you are working on a library management system then you have to work with data related to Books. Fields that will define Book data includes – Title, Author Details (Name of the author, Affiliation of author, Author Address(Alphanumeric field), Mobile Number, Published work and its Number), Number of pages, Date etc. As you can see Book data includes fields having different data types but all the fields are linked to a single entity, Book. To store such data in memory, C language has well defined programming constructs such as structure and union.
In C language similar data type is stored in memory using Arrays but if we want to store non-similar data type then Structure and Union is used. We will study Structure and Union in the following sections.
Structures in C
In C language, structure is defined as follows:
struct structure-name { data_type var-name-1; data_type var-name-2; …. …. …. data_type var-name-n; } ;
Here,
- struct is a keyword defined in C compiler.
- data_type is a built-in data type of C.
- var-name is the name of a variable that will be used to store information.
For example, the structure of a person may include its name, age, salary earned etc. To store information of person in memory following structure will be used:
struct person { int person_id; char name[20]; float salary; };
Structure elements may or may not be stored in contiguous memory blocks and this depends on availability of memory blocks and conditions defined in grammar of C language. Diagrammatic representation of structure may look like this:
struct person
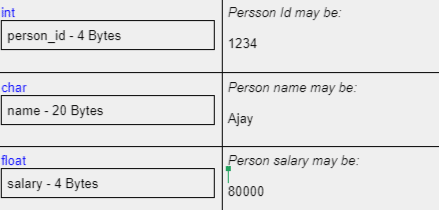
Each element of the structure is called a member of the structure. Members of the structure are accessed using member access operator dot(.). dot operator is used with structure variables. Structure variable is declared as follows:
struct structure_name structure_variable_name;
Here,
- struct is keyword,
- structure _name is name of structure,
- structure _variable_name is name of the structure variable
For example, person structure declared above may have the following structure variable name:
struct person p1;
p1 is the name of the variable that will be used to access structure members using a dot operator like this.
p1.person_id; p1.name; p1.salary; |
C program to implement structure
#include <stdio.h> int main ( ) { struct person { int person_Id; char name [70]; }; struct person per; printf("\n Enter the person_Id"); scanf("%d", &per.person_Id); printf("\n Enter name of person"); scanf("%s", per.name); printf("\n"); printf("Displaying information of person"); printf("\n"); printf("\n Person Id. = %d", per.person_Id); printf("\n"); printf("\n Name = %s", per.name); return 0; }
Output: Enter the person_Id: 1234 Enter name of person: “Ajay” “Displaying information of person” Person Id. = 1234 Name = “Ajay”
Union in C
Union improves memory optimization techniques. Union can contain information of different data types. Amount of memory allocated to Union is the amount of memory allocated to its largest data type. If union is used to declare variables having data types – signed integer (2 byte), signed long int (4 bytes), double (8 bytes) and long double (10 bytes), then the amount of memory allocated to union is 10 bytes. These 10 bytes of memory blocks are overwritten again and again to store information which requires memory of size 1 byte to 10 bytes.
Thus, a single block of memory is allocated to Union and its size depends on the size of the largest data type declared in Union.
Syntax to declare Union:
union union_name { Data type var_name; Data type var_name; …. …. };
Here,
- union is the keyword,
- union_name is the name of the union
- Data type is the in-built C language data type
- var_name is the name of the variable
For example union can be declared as –
union person { int person_Id; Char name[70]; };
Memory block allocated to the union person is 70 bytes. If the programmer wants to store the name of person then this 70 bytes will be used and if the programmer wants to store person_id then this 70 bytes will be overwritten by person Id and only 4 bytes will be used. As it can be understood that, after writing 4 bytes, the remaining 66 bytes will be a wastage of memory.
To access members of Union, dot(.) is used in association with a variable of union
C program to implement Union
#include <stdio.h> int main( ) { union person { int person_Id; Char name[70]; }; union person per; printf("\n Enter the person_Id "); scanf("%d", &per.person_Id); printf("\n Enter name of person "); scanf("%s", per.name); printf("\n"); printf("\n Name = &%s", per.name); return 0; }
Output: Enter the person Id 1234 Enter name of person Ajay Displaying information of person Name = Ajay
Code Analysis From the output of the program it is clear that the name “Ajay” has an overwritten person Id.
Use of Structure and Union in Operating systems
There are many instances where Structure and Union has been used. To understand the importance of structure and union in operating systems, we can take one instance from many where structure and union has been used in operating systems.
Use of structure in Operating System
(as taken from Carnegie Mellon University, School of Computer Science https://www.cs.cmu.edu/~guna/15-123S11/Lectures/Lecture24.pdf):
DIR *opendir(char* dir_name)
This command opens a directory given by dir_name and provides a pointer to access files within the directory. The open DIR stream can be used to access a struct that contains the file information. The function
struct dirent *readddir(DIR* dp)
returns a pointer to the next entry in the directory. A Null is returned when the end of the directory is reached. The struct has the following format.
struct dirent { u-long d_info; u_short d_recien; u_short d_namelen; char d_name[MAXNAMLEN+1]; };
Use of Union in Operating Systems
Union is used in the operating system to pack and unpack data bits in an integer. This allows programmers to store information such as permissions, for everyone else, group permissions, file owner permissions and file data which specifies whether the file is directory or regular file. To store such information following union has been defined in operating system:
union map { Unsigned short statmode; //data input as 16-bit int modes convert; //predefined modes bit field };
map mapper
Here, mapper is a variable of type union map, which means that it can store both unsigned short int and a modes structure, but not at the same time. Union makes operations very fast and is used in Unix, Linux etc.
Structure and Union also has a vital role in Database management systems to store and process records. Both structure and union also have a significant importance in computer networks to store and process data fields.
Key difference between Structure and Union
Structure | Union |
|
|
|
|
|
|
|
|
Leave a Reply