Computer Graphics are generated using display drivers. Display driver instructions are written to interact with graphic hardware called graphic cards. Different graphic cards have different hardware specifications and qualifications to talk to operating systems.
All graphic cards have text mode. Text mode is responsible for generating 256 possible values for graphic characters. Computer graphics also include visual content. Visual content consists of three-dimensional and two-dimensional computer graphics and image processing programs.
Computer graphics represent objects on display monitors by faces, edges and vertices. Faces, edges and vertices are generated using solid modelling. Solid modelling works on mathematical principles and functions.
Mathematical principles and functions are used to define shapes using opaque algorithms. These opaque algorithms are fed with spatial coordinates denoted by x, y on the x-axis and y-axis to generate Vector and Raster type images.
Raster images use digital values, either 0 or 1. These digital values are used by computer hardware to generate intersections of x and y lines to target a specific pixel and connect these pixels to form raster images. On the other hand, Vector images are defined by magnitude or length or direction using points on the Cartesian plane to form polygons and other shapes. Points on the cartesian plane determine stroke colour, shape, curve, thickness and fill. Vector graphics are used to generate SVG, EPS, PDF or AI types of graphic file formats.
To develop an understanding of computer graphic programs, required prerequisites include having cognizant of Applied Mathematics, Computational Geometry, Computational Topology, Computer Vision, Image Processing, Information Visualization and Scientific Visualization.
Computer Graphics in C
Computer Graphics in C language is mostly “Text” mode. To draw graphical shapes like line, rectangle, circle etc., “Graphic” mode is used. To execute the graphic program “graphics.h” header file must be included.
Example of graphics program in C language, this program changes the current background color to required color :
#include <graphics.h> #include<conio.h> int main ( ) { int graph_driver = DETECT, graph_mode; initgraph(&graph_driver, &graph_mode, “ ”); setbkcolor (2); getch(); closegrah(); return 0; }
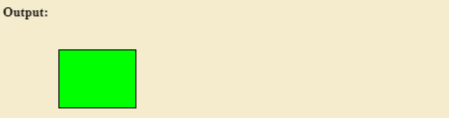
Code Analysis: In the above code, DETECT is macro which auto detect graphic driver graph_driver is related to graphdriver graph_mode is related to graphmode initgraph loads the graphics driver and sets the system into graphics mode in run time setbkcolor (int color) is used to set drawing color to color represented by its integer value having range 0 to 15
Following are the two example functions that are included in <graphics.h> Other graphic functions of C language can be studied at Graphics Program Examples or at Stanford education.
arc
void arc(int x, int y, int stangle, int endangle, int radius)
This function is used to create an arc of a circle.
Here,
- x, y projects center point of arc,
- stangle is an angle at which arc begins,
- endangle is the angle at which arc ends,
- Radius is the radius specifying the height and length of the arc
Example code:
#include <graphics.h> #include<conio.h> int main ( ) { int graph_driver = DETECT, graph_mode; initgraph(&graph_driver, &graph_mode, " ”); arc(100,90,0,135,50); getch ( ); closegraph ( ); return 0; }
bar
void bar(int left, int top, int right, int bottom)
It is used to draw a 2-dimensional, rectangular filled bar.
Here,
left targets top left corner X-coordinate on XY plane,
top targets top left corner Y-coordinate on XY plane,
right targets right bottom corner X-coordinate on XY plane,
bottom targets right bottom corner Y-coordinate on XY plane.
Example code:
#include <graphics.h> #include<conio.h> int main ( ) { int graph_driver = DETECT, graph_mode; initgraph(&graph_driver, &graph_mode, “ ”); bar(80,90,190,350); getch ( ); closegraph ( ); return 0; }
Hardware Usage in Graphics Programming
Graphic programming instructions are complex. These instructions interact with multiple hardware components before generating graphics on computer displays. Code complexity of graphics programs can be understood by considering the logic of generating a filled square, filled circle etc. To draw a filled bounded square or circle we have to connect pixels using x and y coordinate over the display grid and since multiple pixels have to be connected repeatedly it requires iteration. Iteration is achieved using loops. Use of a large number of loops increases code execution complexity and bound programmers to consider hardware performance factors.
Graphic programming instructions interact with multiple hardware such displays, keyboard inputs, Arithmetic Logic Unit (ALU), pipeline hardware, control unit, plus L1 instruction and data caches giving rise to the concept of parallelism and complexity. Graphic programming instructions executed in parallel attains high throughput. To achieve instruction level parallelism work load in each CPU clock cycle is increased.
In the 1980’s the first digital computer was used in graphic programming but its cost and maintenance was too high due to which its use was restricted to commercial settings only. Firms like Macintosh worked on computer hardware to reduce its cost and bring it into the range of personal computers. In and around 1980, Macintosh and other research labs exploited Pipelining, Superscalar Techniques, and Simultaneous Multithreading (SMT) technology using which high frequency execution of instructions per CPU clock cycle was achieved and that too at low cost.
In order to achieve an uninterrupted output of a computer graphics program on computer displays, CPU’s must be chosen that supports high processor logic against simple memory logic.
Final Words
Computer graphic programming is used to draw pictures, lines, charts etc., using pixels. Images on screen are generated using Vector Scan and Raster Scan. To generate images on screen, information about magnitude and direction of pixels is required. This information is fed into mathematical functions to draw an image. To achieve fast execution, parallel execution of mathematical functions must be done and must maintain a balance between used hardware configuration.
To learn Graphics Programming in C language, a programmer must develop an understanding of the following algorithms:
- DDA Algorithm
- Bresenham’s Line Drawing Algorithm
- Bresenham’s Circle Drawing Algorithm
- Bresenham’s Ellipse Drawing Algorithm
- Two-Dimensional Transformation
- Composite Two-Dimensional Transformation
- Cohen Sutherland 2D Line Clipping
- Windowing to viewport mapping
- Polygon Clipping Algorithm
- Three-Dimensional Transformation
- Composite Three-Dimensional Transformation
- Visualising Projections of 3D Images
Using these algorithms and C programing constructs, moveable and unmoveable objects on the computer screen can be generated
Leave a Reply