DOS is a single tasking operating system that means only one application program can be executed at a time. Program execution in DOS is sequential or we can say DOS is a batch processing operating system. If the program execution fails, the DOS operating system goes into a deadlock state and we say that system is freezed.
In Windows operating systems multiple applications can be executed simultaneously, this is known as multitasking Operating System. Multitasking feature is possible by interrupting the flow of execution control. Flow execution control can be interrupted with the help of branch and jump instructions.
Different programming languages offer different types of branch and jump instructions. C language has 3 types of instructions. These are:
- Type declaration
- Arithmetic
- control
Type declaration instructions:
Using Type declaration instruction variable in C language are declared for example,
int a = 5;
Arithmetic instructions:
C language supports 3 types of arithmetic instructions, as follows:
- Integer mode
- Real mode
- Mixed mode
Control instructions:
C language has four types of control instructions.
- Sequence instruction
- Decision instructions
- Repetition instructions
- Case instructions
Jumping and Branching
C Language is used to develop application programs. Execution of instructions in a program is sequential for example if a program has 3 instruction sets – instruction set A, instruction Set B, and instruction Set C. In this case instruction set A is executed first, then instruction Set B is executed and at last instruction Set C is executed. This is an example of sequential execution of instructions.
In programming, it is often required to execute instructions in non sequential order. For example, after executing instruction set A, instruction Set C is executed as we can see that execution of instruction set B is skipped. This is an example of non-sequential execution order or we can say that this is an example of branching and jumping. To execute instructions in non sequential order branching and jumping is required. C language has different types of branching and jumping instructions.
Branching in C language
Branching in C language is possible with the help of if-else statements.
If-else statement
If-else statement is also known as a decision control statement. Syntax of if-else statement is as follows:
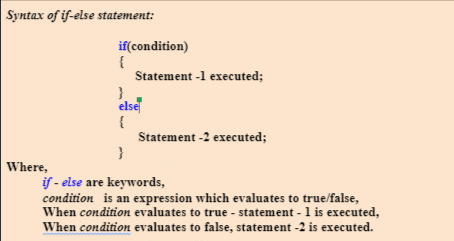
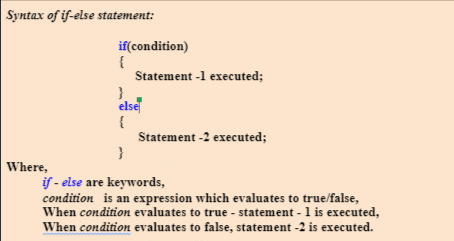
//Example program of if-else statement
#include <stdio.h> int main(void) { int a=20,b=10; if(a>b) { printf("a is greater"); } else { printf("b is greater"); } return 0; }
Output: a is greater.
Code Analysis In the above code relation condition a>b is evaluated. Variable ‘a’ has value 20 which is compared with variable ‘b’ having value 10, making condition a>b (20>10), this condition evaluates to true resulting in the execution of printf(“a is greater”).
Nested-if statement
Nested if means if statement inside another if statement. Syntax of nested-if statement is:
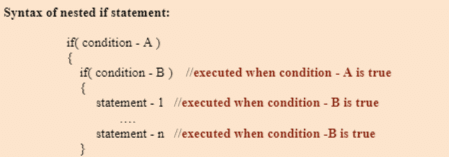
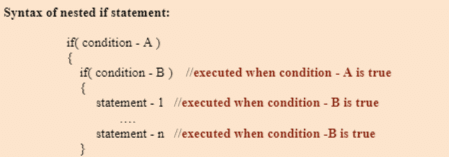
#include <stdio.h> int main(void) { int a = 20, b = 10, c = 3; if(a>b) { if(b>c) { printf("a is greater"); } } return 0; }
Output: a is greater
Code Analysis: In the above code two conditions are checked. First condition, a>b is associated with the first if statement. If the first condition is evaluated to be true then the second condition b>c is evaluated. Second condition is associated with the second if statement which is within the execution block of the first if statement. Above code is an example of nested if statements.
If-else exercise:
Exercise-1
# write a C program to find the second largest number in the array.
#include <stdio.h> void q_sort(int init_arr[11], int lh, int rh); int part (int init_arr[11], int lh, int rh); int main ( ) { int p, ps = 0; int init_arr[10] = {12, 25, 45, 121, 110, 128, 2, 617, 0, 6 }; int sec_lar[10]; printf(" "); printf("\n"); printf("\t \t \t \t"); printf("\n"); printf("\n"); printf("Array elements are"); printf(" "); printf("\n"); for(p=0; p<10;p++) { printf("\t"); printf("%d", init_arr[p]); } printf(" "); printf("\n"); q_sort(init_arr, 0, 9); printf(" "); printf("\n"); printf("Second largest number in the array"); printf(" "); printf("\n"); for(p=9; p>=0; p--) { sec_lar[ps] = init_arr[p]; ps++; } printf("%d", sec_lar[1]); return 0; } void q_sort(int init_arr[10], int lh, int rh) { if(lh<rh) { int til =part(init_arr, lh, rh); if(til>1) { q_sort(init_arr, lh, til-1); } if( til+1<rh) { q_sort(init_arr, til+1, rh); } } } int part(int init_arr[10], int lh, int rh) { int til = init_arr[lh]; while(1) { while(init_arr[lh] < til) { lh++; } while(init_arr[rh]>til) { rh--; } if(lh<rh) { if(init_arr[lh] == init_arr[rh]) { return rh; } int tempo = init_arr[lh]; init_arr[lh] = init_arr[rh]; init_arr[rh] = tempo; } else { return rh; } } }
Output: Array elements are: 12, 25, 45, 121, 110, 128, 2, 617, 0, 6 Second largest number in the Array is: 128
Exercise -2
Using if-else condition write a C program to convert lowercase character to uppercase character
#include <stdio.h> #include<string.h> int main( ) { char L_U[100], U[100]; int ii, count; printf(“\n Enter a string ”); scanf(“%[^\n]”, L_U); count = strlen(L_U); for(ii=0; ii<count; ii++) { if(L_U[ii] >= ‘a’ && L_U[ii] <= ‘Z’) { U[ii] = (char) (L_U[ii] - ‘a’+’A’); } else { U[ii] = L_U[ii]; } } printf(“\n”); for(ii=0; ii<count; ii++) { printf(“%c”, U[ii]); } return 0; }
Output: Enter a string This is boy THIS IS BOY
Exercise – 3
# Using jumping and branching, write the Dijkstra program in C.
#include<stdio.h> #include<limits.h> typedef enum {false, true} bool; void dijkstra_algo(int g_raph[9][9], int source, int vertices_Count); int minimum_distance(int d_istance[40],bool shortest_path_tree_set[9], int vertices_Count); void p_rint(int d_istance[40], int vertices_Count); int main( ) { int grd[9][9]= { {1,6,10,20,2,30,40,19,50}, {3,40,4,20,50,4,20,1,60}, {40,67,40,12,10,23,30,20,56}, {10,60,25,30,19,116,70,80,90}, {40,30,20,29,10,10,40,50,70}, {20,40,62,60,120,50,22,21,80}, {40,40,50,116,20,32,40,21,16}, {59,141,60,20,60,20,171,50,85}, {60,80,32,40,60,70,64,75,40} }; digikstra_algo(g_raph,0,9); return 0; } int minimum_distance(int d_istance[40], bool shortest_path_tree_set[9], int vertice_Count) { int v; int min = INT_MAX; int min_Index = 0; for(v=0;v<vertice_Count;++v) { if (shortest_path_tree_set[v]==false && d_istance[v]<=min) { min = d_istance[v]; min_Index = v; } } return min_Index; } void p_rint(int d_istance[ ], int vertices_Count) { int i; printf(“Distance of Vertex from source is: \n”); for(i=0;i<vertices_Count;++i) { printf(“%d \t %d \n”,i, d_istance[i]); } } void dijkstra_algo(int g_raph[9][9], int source, int vertices_Count) { int count,v,i,j; int d_istance[40]; bool shortest_path_tree_set[9]; for(i=0;i<vertices_Count;i++) { d_istance[i]=INT_MAX; shortest_path_tree_set[i]=false; } d_istance[source]=0; for(count=0;count<vertices_Count-1;++count) { int u = minimum_distance(d_istance, shortest_path_tree_set, vertices_Count); printf(“Minimum Distance value is %d\n”,u); shortest_path_tree_set[u] = true; for(v=0;v<vertices_Count;++v) { printf(“\n”); printf(“the value of v %d”, v); printf(“\n”); if(!shortest_path_tree_set[v]) { printf(“I am in !shortest_path_tree_set[v] if statement \n”); if(d_istance[u]!=INT_MAX) { printf(“I am in d_istance[u]!=INT_MAX if statement \n”); printf(“%d \n”, g_raph[u][v]); printf(“d_istance[v] %d \n”, d_istance[v]); printf(“d_istance[source] %d \n”, d_istance[source]); printf(“d_istance[u] %d \n”, d_istance[u]); printf(“d_istance[u]+g_raph[u][v] %d \n”, d_istance[u]+g_raph[u][v]); if( d_istance[u]+g_raph[u][v] < d_istance[v] ) { printf(“I am in d_istance[u]+graph[u][v]<d_istance[v] If statement \n”); d_istance[v]=d_istance[u]+g_raph[u][v]; printf(“d_istance[v] %d \n”, d_istance[v]); } } } } p_rint(d_istance,vertices_Count); }
Output: Distance of vertex from source is: 0 0 1 6 2 10 3 20 4 2 5 10 6 26 7 7 8 42
Leave a Reply