System programming is the process of constructing instructions using which computer hardware can interact with computer programmers and the user. Hierarchy of system program is as follows:
- Operating System
- Compilers
- Assemblers
- I/O routines
- Interpreters
- Scheduler
- Loaders and Linkers
System programming is hardware dependent. To develop an efficient system program, knowledge of computer hardware is must as system programs are platform dependents.
In system programming, programs are written in low level language using language such as C, C++ and C#.
Low-level programs developed in system programming interact with computer hardware accessing register and memory locations. The system programs of operating systems consists of Memory management, CPU scheduling, Device handling programs etc, these System programs are processed by Assembler.
Assembler in System Programming
An assembler converts assembly language code into machine language code. Assembler also generates instructions for the loader such as externally defined symbols. Task of an assembler can be best understood with the help of the program. Example steps in assembling a program is given in Table 1:
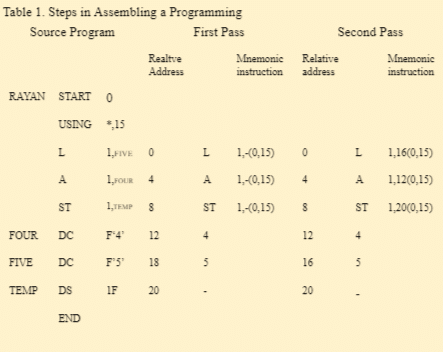
In Table 1 RAYAN is the name of the program. The name “RAYAN” is passed to the loader. The next instruction is the USING *,15 it means that 15 is the base register and will point to the beginning of the program. Next is, load instruction L 1, FIVE. Next is Add instruction A 1, FOUR. DC instruction is used to define data DC F ‘4’ that means 4 is stored at relative location 12. It also means that the first instruction is four bytes long. Next instruction DC F ‘5’ has label FIVE having location counter 16. Next instruction DS 1F has label TEMP having value 20.
An assembler carries out two passes: in the first pass symbols are defined and in the second pass instructions and addresses are defined. An assembler can be one pass or multiple pass.
Following are the functions carried out by assembler:
- Produce Instructions by:
- Generating machine code
- Finding symbol value, performing operations on literals and allocating addresses.
- Working-out pseudo operations
Logically these operations are grouped into two passes. These are:
Pass 1: Objective – to define symbols and literals
- To find machine instructions length.
- Recording location counter values.
- Recording symbol values.
- Working with pseudo operations.
- Recording literals.
Pass 2: Objective – to produce object program
- Finding symbol values.
- Producing instructions.
- Produce data.
- Working-out pseudo operations
Data structure used in Pass 1 and Pass 2 of assembler are:
Pass 1:
- Source program is fed.
- Location counter is used to keep the address of instruction.
- Machine-Operation Table keeps record of symbols and its length.
- Pseudo-Operation Table indicates action to be performed for each pseudo operation.
- Symbol Table (ST) records the value of a symbol and its value.
- Literal Table records literal used in program and its value.
- A generic copy of input.
Pass 2:
- Source program copy.
- Location counter
- Machine Operation Table to indicate instructions
- Pseudo-Operation Table to indicate symbols and desired actions
- Symbol Table to record symbols and values
- Base Table to record registers acting as Base registers
- PRINT LINE to generate list
- PUNCH CARD generates instructions in the format that are acceptable by loader.
- Arranging instructions in loader acceptable format.
Assemblers use different types of algorithms to perform searches on its data structure. Assemblers perform linear search to find values of symbols used. When the value of symbols has to be retrieved from a table whose entries are not sorted then in that case assembler uses binary search.
When the symbol table maintained by assembler is sorted then assembler uses binary search. Binary search is more efficient and effective than linear search. Binary search can perform search operations on the symbol table in log2 N time. To perform binary search, the symbol table needs to be sorted. To perform sorting, different algorithms are used by the assembler. Sorting algorithm used by assembler are – Interchange Sort, Shell Sort, Bucket sort, Radix exchange sort and address calculation sort.
Leave a Reply