Following is the structure of C program:
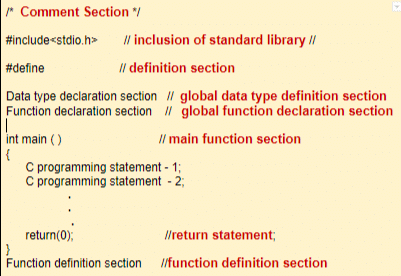
C program structure consists of following section:
- Comment Section
- Inclusion of standard library
- Definition Section
- Global data type declaration section
- Global function declaration section
- Main function section
- Function definition section
Comment Section: Comment section can be used to provide program metadata. The metadata includes details about the program such as the author of the program, date of creation of the program, brief description of the program such as what it is about, association of this program with other programs and if the program requires specific input details about it.
Example of comment section:
/*
File Name: <name of the file>.c e.g. sample.c
Author: DiRk
Date: 10/4/2021
Description: this is a sample program. It requires no special input.
*/
- Inclusion of Standard library section: This section is also known as header file section or preprocessor directory. Header file section or preprocessor directory section is used to specify the name of the files required to run a C program. Header file section gives you the name of the header file to be included in the program. Before the execution of the program begins the header file must be included in the program.
The compiler produces the object code. The object code consists of the program developed by the programmer as well as the program given in the header file.
Header files are used to reduce the complexity of the code as well as to achieve the functionality of reusability. Once a program is defined as a preprocessor directory it can be used multiple times and in different programs simultaneously.
For example printf ( ) function is used to display output of the program and scanf ( ) functions are used to take input from the user. These functions belong to the standard library of the C compiler. Definition of this function is the programming instructions required to take input form the user and display output to the user are given in the standard library file named “stdio.h” it means standard input output.
Table 1 gives the header files supported by the standard C language compiler:
Table 1 Header files
ALLOC | ASSERT | BCD | BIOS | COMPLEX | CONIO |
CONSTERA | CTYPE | DIR | DIRECT | DIRENT | DOS |
ERRNO | FCNTL | FLOAT | FSTREAM | GENERIC | GRAPHICS |
IO | IO,AMIP | IOSTREAM | LIMITS | LOCALE | LOCKING |
MALLOC | MATH | MEM | MEMORY | NEW | PROCESS |
SEARCH | SETJMP | SHARE | SIGNAL | STDARG | STDDEF |
STDIO | STDIOSTR | STDLIB | STRING | STRSTREA | TIME |
UTIME | VALUES | VARARGS |
- Definition section: Definition section is used to define constants and macros. The keyword define is used to declare constants or macros. define is used as follows:
#define PI = 3.14
C Macros is a set of programming instructions to be replaced by its macro name. Macro is declared by #define directive. C language supports these two types of Macros – Object-like Macro and Function-like Macro.
When Object-like macros are used programming instructions are replaced by its value. It is used to declare programming constants.
Function-like macros work like functions. C language support following predefined functions:
- _Date_ : it represents the date.
- _TIME_: it represents time.
- _File_ it represents file name.
- _LINE_: it represents line number.
- _STDC_: its value is 1 for ANSI standard.
Example of definition sections are used for constant:
#define P=4.53
Example of definition section when used for Macro as a function:
#define MINMAX(a,b)((a)<(b)?(a):(b))
Global declaration section: In this section variables that will be used in all the defined functions of the program are declared. This section is also used to declare functions, structures and unions.
Variables declared in functions are local in scope. Local can not be used outside its scope. To use the variable throughout the program global variables are used. When variables are declared outside the scope of functions building up the program then the compiler treat it as a global variable.
Global variables are declared at the beginning of the program before the declaration of the functions.
Example global variable is like this:
#include<stdio.h> #define p = 4.56 int k =5; void sum( ); int main( ) { int j =3; int s = 0; s = j+k; sum( ); } void sum( ) { printf(“The value of sum is %d”, k); }
Global section is also used to declare structure and union. Structure/Union in C language is declared as follows:
#include<stdio.h> #define P=3.56 int k = 67; struct std { int a; int b; } alpha; void sum( ); int main( ) { alpha. a = 5; alpha.b = 6; sum( ); } void sum( ) { int j; int s = 0; j= k+s; }
- main( ) function: Execution of C program begins with main(). Programming instructions are written in main( ). In C language it is required that all the local variables that will be used within the program should be declared at the beginning of the program. Thus, the main() function begins with the variable declaration. After variable declaration programming instructions are written to obtain desired results.
The opening of the main( ) function is marked by this curly bracket “{” and closing is marked by this “}” bracket.
- Function definition section: When the number of programming instructions is large it decreases reliability and increases the difficulty in understanding the logic of the program. Thus, it becomes the requirement to subdivide the program according to its functionality.
Functions have specific purposes and needs. In C language, functions must be declared in the global section before main( ). Declaration of the function must be done before it is used. Functions are called from the main( ) or within the function. Definition of the function can be given before main ( ) or after main ( ). When the execution of the function completes control will be returned to the next line from where the function is called.
Example of function and main ( ) is given below:
#include<stdio.h> #define P=3.56 int k = 67; struct std { int a; int b; } alpha; void sum( ); int main( ) { alpha. a = 5; alpha.b = 6; sum( ); } void sum( ) { int j; int s = 0; j= k+s; }
Leave a Reply