What is Builder Factory Design Pattern?
- It falls under the Creational Design Pattern.
- The term “Builder” itself conveys the notion of “building something”.
- In the case of programming, Build Design Pattern falls under Creational Design Patterns which is used to build complex objects using simpler ones.
Table of Contents
Book Definition of Builder Design Pattern
It separates the construction of a complex object from its representation so that the same construction process can create different representations.
Real Life Examples of Builder Design Pattern
Example 1:
If we consider a house, we need to go through a step by step procedure. First, we need to set the basement. Next comes the pillars. Then you’ll need bricks and cement etc.
For building up a house, you’ll need a combination of stuff like bricks, rod, iron rod, cement, and many other things. Similarly, for creating a complex Java object, you need any number of smaller and simpler objects.
Example 2:
For laptop manufacturing purpose, there are several steps included like setting the memory hard disk and then LCD skins and then the number of other steps. After completion of these steps, a laptop as a final object can be created.
Example 3:
For beverages like Coffee, Boost or Horlicks, you need to follow some steps generically. First, you’ll add water, then milk and sugar. Then based on our requirement, we’ll choose whether we need to add Coffee, Horlicks or Boost powder as our final ingredient. These are the simple steps with simple ingredients for making a complex drink like a beverage.
Example 4:
In the case of Pizza making, first, you’ll need a dough as the base object. Then you’ll need to add layers of cheese, vegetables, meat or anything based on requirement. Here, vegetables, and cheese, etc. are simple objects that are combined to make a Pizza.
UML Class Diagram of Builder Design Pattern
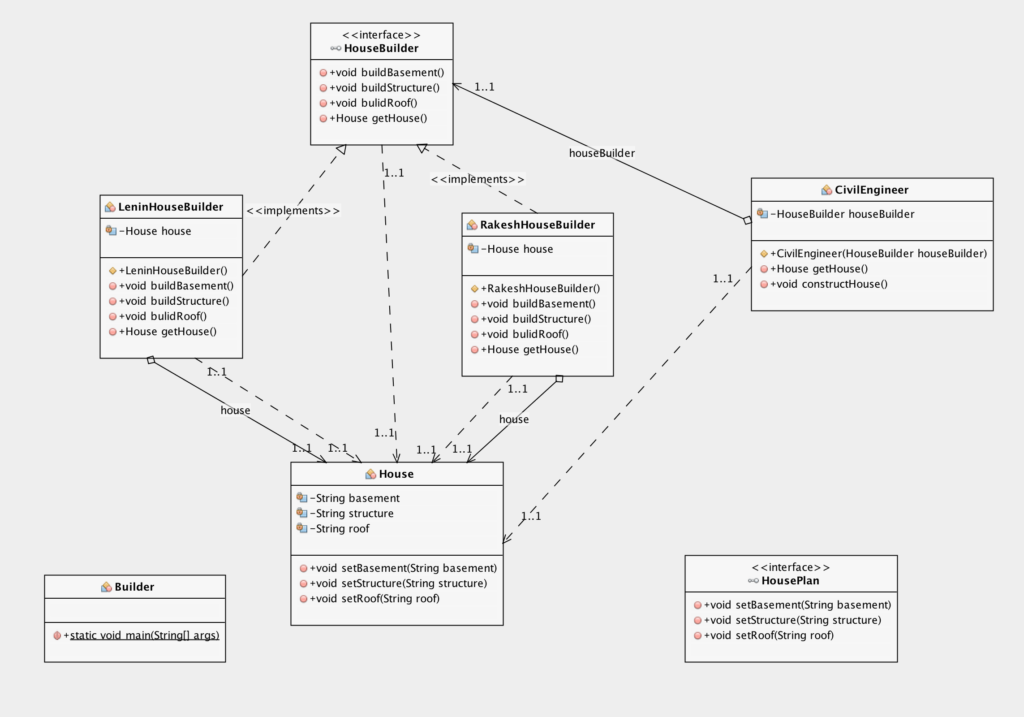
Builder Design Pattern Java Implementation
- House construction:
- Consider “Rakesh HouseBuilder’, “Lenin House Builder”, are two types of house builders for construction a “House”.
- To create complex objects like “House”, we need to follow the generic steps.
- Step 1: Set the basement.
- Step 2: Build pillars.
- Step 3: Set the roof.
- Step 4: Do interior work
- Step 5: etc.
- Step 6: Finally, we have created a complex object called “House”
Step 1: First create an interface "HousePlan"
public interface HousePlan { public void setBasement(String basement); public void setStructure(String structure); public void setRoof(String roof); }
Step 2: Create a class to hold "House" attributes
public class House { private String basement; private String structure; private String roof; public void setBasement(String basement) { this.basement = basement; } public void setStructure(String structure) { this.structure = structure; } public void setRoof(String roof) { this.roof = roof; } }
Step 3: Create a HouseBuilder Interface
public interface HouseBuilder { public void buildBasement(); public void buildStructure(); public void bulidRoof(); public House getHouse(); }
Step 4: Create a class "RakeshHouseBuilder" which implements "HouseBuilder"
public class RakeshHouseBuilder implements HouseBuilder { private House house; public RakeshHouseBuilder() { this.house = new House(); } @Override public void buildBasement() { house.setBasement("First class sand"); } @Override public void buildStructure() { house.setStructure("Cement block"); } @Override public void bulidRoof() { house.setRoof("Cement Roof"); } @Override public House getHouse() { return this.house; } }
Step 5: Create a class "LeninHouseBuilder" which implements "HouseBuilder"
public class LeninHouseBuilder implements HouseBuilder { private House house; public LeninHouseBuilder() { this.house = new House(); } @Override public void buildBasement() { house.setBasement("Second class sand"); } @Override public void buildStructure() { house.setStructure("Wood block"); } @Override public void bulidRoof() { house.setRoof("Wooden Roof"); } @Override public House getHouse() { return this.house; } }
Step 6: Create a CivilEngineer class which uses HouseBuilder to build a House
public class CivilEngineer { private HouseBuilder houseBuilder; public CivilEngineer(HouseBuilder houseBuilder) { this.houseBuilder = houseBuilder; } public House getHouse() { return this.houseBuilder.getHouse(); } public void constructHouse() { this.houseBuilder.buildBasement(); this.houseBuilder.buildStructure(); this.houseBuilder.bulidRoof(); } }
Step 7: Create a main class
public class Builder { public static void main(String[] args) { HouseBuilder rakeshBuilder = new RakeshHouseBuilder(); CivilEngineer engineer = new CivilEngineer(rakeshBuilder); engineer.constructHouse(); House house = engineer.getHouse(); System.out.println("House constructed: "+house); } }
Leave a Reply